OOPS interfaces¶
All OOPS applications interact with specific model- or observation- related implementations through interface classes. There are two kinds of interface classes, described below.
Interface classes chosen at build time¶
OOPS applications and classes are templated on OBS and/or MODEL traits. These traits are C++ structures defining which of the interface class implementations should be used in the current class or application.
OBS
traits contain interface classes that are related to the observation processing:
ObsSpace
: observation data handling for one obs type;ObsVector
: observation vector (e.g. vector of observation values, or of computed H(x));ObsDataVector
: templated on data type vector in observation space (oops currently usesObsDataVector<int>
andObsDataVector<float>
);ObsOperator
: observation operator, used for computing H(x);LinearObsOperator
: tangent-linear and adjoint of the observation operator;ObsAuxControl
: auxiliary state related to observations (currently only used for bias correction coefficients, but can be used for other cases);ObsAuxIncrement
: auxiliary increment related to observations (currently only used for bias correction coefficient increments);ObsAuxCovariance
: auxiliary error covariance related to observations (currently only used for bias correction coefficient error covariances);ObsAuxPreconditioner
: preconditioner for auxiliary error covariance related to observations (currently only used for the preconditioning of the bias correction coefficient error covariances);Locations
: observations locations, used in the interface between model and observations, for interpolating model fields to observations locations to be used in H(x);GeoVaLs
: geophysical values at locations: model fields interpolated to the observation locations; used in H(x);ObsDiagnostics
: observation operator diagnostics: additional output from the observation operator that can be used in quality control.
There are currently three implementations of OBS
traits in JEDI: lorenz95::L95ObsTraits
used with the toy Lorenz95 model, qg::QgObsTraits
used with the toy quasi-geostrophic model, and ufo::ObsTraits
used with all realistic models connected to JEDI. ufo::ObsTraits
lists classes implemented in ioda and ufo as the implementations of above interfaces. All the new models added to JEDI can make use of ufo::ObsTraits
and do not have to implement any of the above classes.
MODEL
traits contain interface classes that are related to models:
Geometry
: model grid description, MPI distribution;State
: analysis state, model state, ensemble member, etc;Increment
: perturbation to a state;LocalInterpolator
: optional, interpolator from/to state locations to/from specified locations on the same MPI task. IfLocalInterpolator
is not specified in theMODEL
trait, a genericoops::UnstructuredInterpolator<MODEL>
will be used as a local interpolator;VariableChange
: variable change in the model space;LinearVariableChange
: tangent-linear and adjoint of the variable change;ModelAuxControl
: auxiliary state related to model (currently not used, could be e.g. model bias);ModelAuxIncrement
: auxiliary increment related to model;ModelAuxCovariance
: auxiliary error covariance related to model;ErrorCovariance
: background error covariance (optional);GeometryIterator
: iterator over model grid points (only used in theLocalEnsembleDA
applications);NormGradient
: scale an increment (gradient) according to a given norm (only used inAdjointForecast
).
Classes in the MODEL
traits are typically implemented for each distinct model used in JEDI. Depending on the oops application, only a subset of these classes may have to be implemented.
Example of OOPS implementation of classes defined in traits¶
Fig. 1 shows class diagram for the Geometry
class.
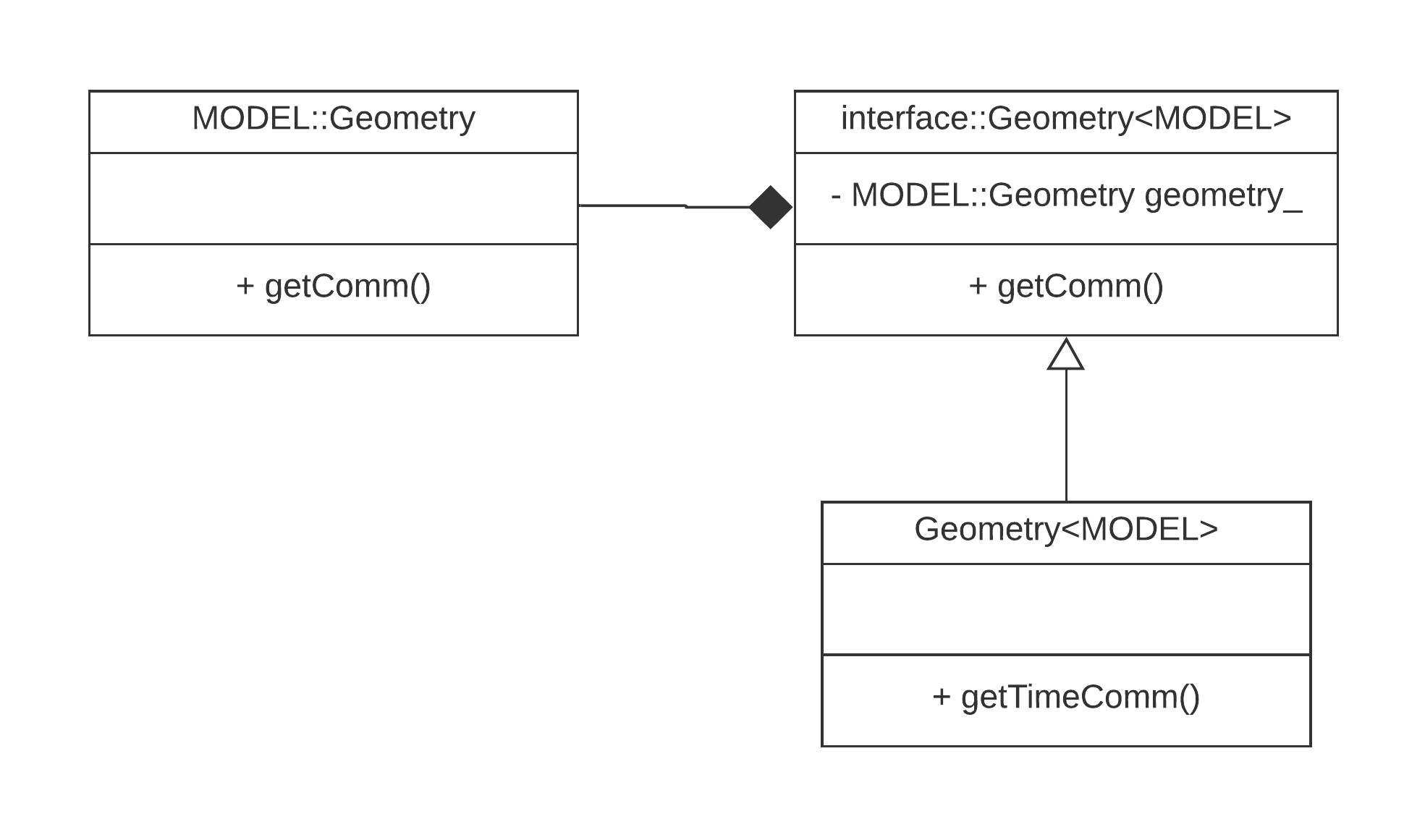
Fig. 1 Class diagram for Geometry-related classes¶
Here MODEL::Geometry
implementation (an implementation of Geometry
specified in the MODEL
traits, e.g. Geometry implemented in Lorenz95 model, or fv3-jedi model, or mpas-jedi model) has to implement the interface defined in interface::Geometry<MODEL>
class (in the interface
directory in oops). interface::Geometry<MODEL>
holds a pointer to MODEL::Geometry
implementation; each of the methods in interface::Geometry<MODEL>
calls a corresponding method in MODEL::Geometry
(with added tracing and timing). If any of the interface::Geometry<MODEL>
methods took SomeClass<MODEL>
on input, MODEL::SomeClass
would be passed during the call to the MODEL::Geometry
implementation of the method.
Geometry<MODEL>
(in the base
directory in oops) is a class that is used in oops classes interacting with geometries. It is a subclass of interface::Geometry<MODEL>
that has additional functionality that is implemented at oops level (and doesn’t have to be implemented in MODEL
implementations).
Note
Not all the interface classes are implemented with interface::Class
and Class
distinction yet.
Note
For the interface classes that do not need additional functionality implemented at oops level (e.g ObsOperator
, VariableChange
), there is no need for interface::Class
and Class
distinction. In this case Class
interface class is located in interface
directory.
Interface classes chosen at runtime¶
For classes in this category, there could be multiple implementations of the class, both generic and specific. Generic implementations are templated on the MODEL
and/or OBS
trait and can be instantiated for any (or at least multiple) implementations of these traits. MODEL
- and OBS
-specific implementations are not templated on MODEL
or OBS
– they are tied to a specific implementation of these traits. The classes in this category are typically not defined in OBS
or MODEL
traits, and the class used in each particular case is decided at runtime, based on provided configuration file.
Some of the classes that are currently in this category: Model
, ObsError
, ObsFilter
.
Fig. 2 shows class diagram for the Model
class.
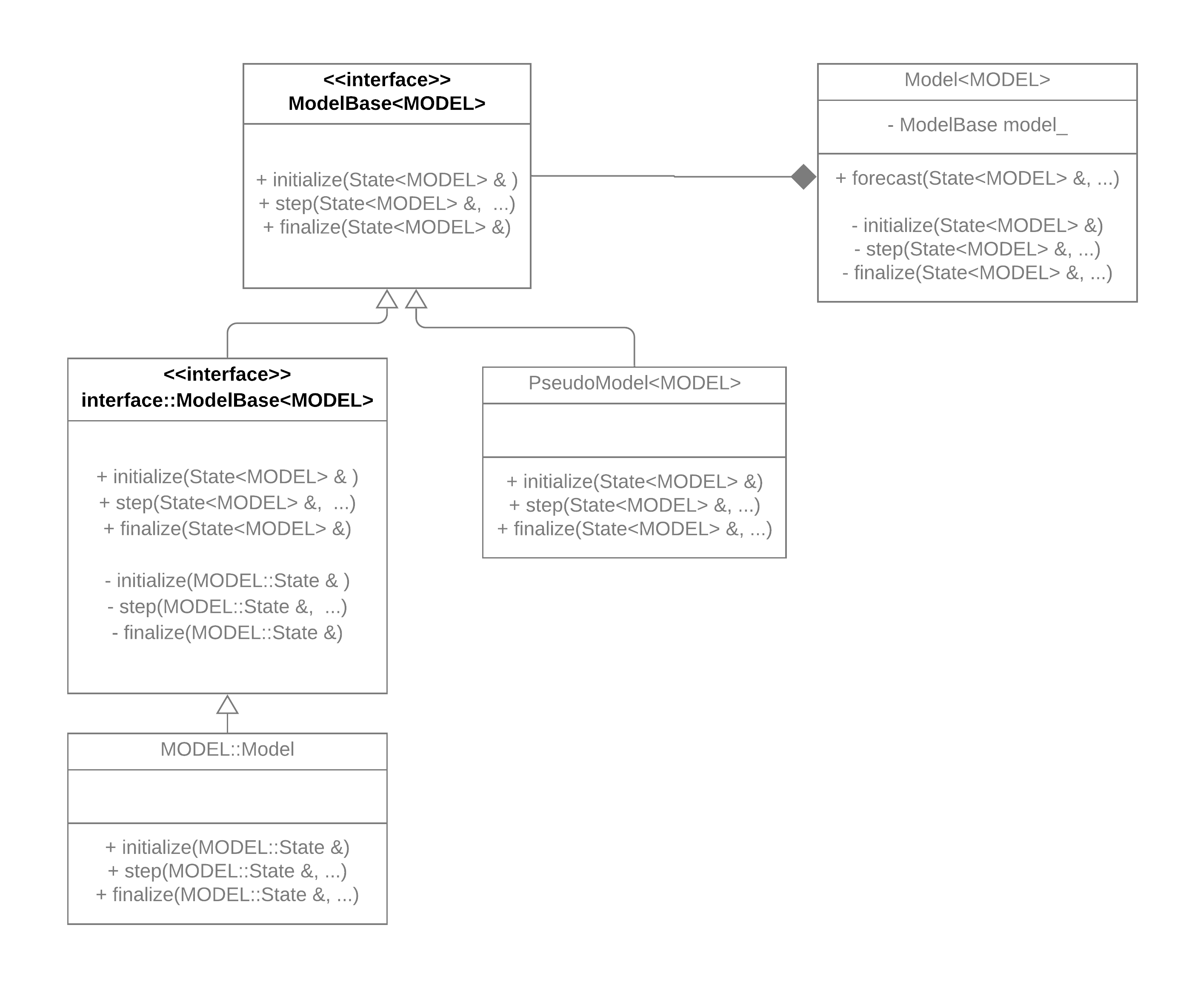
Fig. 2 Class diagram for Model-related classes¶
ModelBase<MODEL>
class methods have templated parameters (e.g. State<MODEL>
in the example). Its subclasses, generic implementations of Model
, can operate on multiple implementations of MODEL
traits (e.g. PseudoModel<MODEL>
which “propagates” model by reading precomputed states from files). Both ModelBase<MODEL>
and generic implementations of Model
reside in generic
directory in oops.
interface::ModelBase<MODEL>
(in the interface
directory) is a subclass of ModelBase<MODEL>
that overrides all methods that take templated parameters with calls to the abstract methods that take parameters tied to a specific implementation of the traits (e.g. MODEL::State
in the example). Subclasses of interface::ModelBase<MODEL>
are not templated on MODEL
and are tied to specific implementations of MODEL
trait (e.g. Lorenz95 prognostic model, FV3-GEOS, FV3-GFS, etc).
Model<MODEL>
(in the base
directory) holds a pointer to ModelBase<MODEL>
. It has methods that correspond to all the methods in ModelBase<MODEL>
; each of those methods calls a corresponding method in ModelBase<MODEL>
(with added tracing and timing). There is also additional functionality in Model<MODEL>
that is included at oops level (and doesn’t have to be included in the implementations), e.g. forecast
method.
Note
Not all the interface classes in this category are implemented according to the above class diagram yet, but the intention is to implement all of them in a similar way.